Lab - 3 6502 Math and Strings Lab
Assembly language is a low-level programming language used to interface directly with the hardware of a computer.
For this I have to create a program that fits the following criteria:
- Your application must run in the 6502 Emulator.
- You must output to both the character screen and the graphics (bitmapped) screen.
- You must take user input from the keyboard in some way.
- You must employ some arithmetic/math instructions (to add, subtract, do bitwise operations, or rotate/shift).
I'll go with Kaleidoscope because I remember playing it when I was younger and have a rough concept of how it works. The code I'm writing allows the user to draw pixels in any of several distinct colours on one-quarter of the screen. In addition, the code allows the user to mirror the pixel to the other three quadrants. A pixel drawn at (0,0), for example, should be mirrored to (31,31), (0,31), and (0,31). (31, 0).
Here is the source code for this game that I wrote:
; zero-page variable locations define ROW $20 ; current row define COL $21 ; current column define POINTER $10 ; ptr: start of row define POINTER_H $11 ; constants define DOT $01 ; dot colour location define CURSOR $05 ; green colour setup: lda #$0f ; set initial ROW,COL sta ROW sta COL LDA #$01 STA DOT draw: jsr draw_cursor getkey: lda $ff ; get a keystroke ldx #$00 ; clear out the key buffer stx $ff cmp #$30 bmi getkey cmp #$40 bpl continue SEC sbc #$30 tay lda color_pallete, y sta DOT jmp done continue: cmp #$43 ; handle C or c beq clear cmp #$63 beq clear cmp #$80 ; if not a cursor key, ignore bmi getkey cmp #$84 bpl getkey pha ; save A lda DOT ; set current position to DOT sta (POINTER),y jsr draw_on_quads pla ; restore A cmp #$80 ; check key == up bne check1 dec ROW ; ... if yes, decrement ROW jmp done check1: cmp #$81 ; check key == right bne check2 inc COL ; ... if yes, increment COL jmp done check2: cmp #$82 ; check if key == down bne check3 inc ROW ; ... if yes, increment ROW jmp done check3: cmp #$83 ; check if key == left bne done dec COL ; ... if yes, decrement COL clc bcc done clear: lda table_low ; clear the screen sta POINTER lda table_high sta POINTER_H ldy #$00 tya c_loop: sta (POINTER),y iny bne c_loop inc POINTER_H ldx POINTER_H cpx #$06 bne c_loop done: clc ; repeat bcc draw draw_cursor: lda ROW ; ensure ROW is in range 0:31 and #$0f sta ROW lda COL ; ensure COL is in range 0:31 and #$0f sta COL ldy ROW ; load POINTER with start-of-row lda table_low,y sta POINTER lda table_high,y sta POINTER_H ldy COL ; store CURSOR at POINTER plus COL lda #CURSOR sta (POINTER),y rts draw_on_quads: LDA POINTER ;; save the pointer to the PHA ;; original location in top_left_quad LDA POINTER_H PHA LDA #$10 CLC SBC COL CLC ADC #$10 TAY LDA DOT STA (POINTER),y TYA PHA ; save the y offset lda #$10 ; load POINTER with start-of-row CLC SBC ROW CLC ADC #$10 TAY lda table_low,y sta POINTER lda table_high,y sta POINTER_H ldy COL ; store CURSOR at POINTER plus COL lda DOT sta (POINTER),y PLA TAY lda DOT sta (POINTER),y PLA STA POINTER_H PLA STA POINTER RTS ; these two tables contain the high and low bytes ; of the addresses of the start of each row table_high: dcb $02,$02,$02,$02,$02,$02,$02,$02 dcb $03,$03,$03,$03,$03,$03,$03,$03 dcb $04,$04,$04,$04,$04,$04,$04,$04 dcb $05,$05,$05,$05,$05,$05,$05,$05, table_low: dcb $00,$20,$40,$60,$80,$a0,$c0,$e0 dcb $00,$20,$40,$60,$80,$a0,$c0,$e0 dcb $00,$20,$40,$60,$80,$a0,$c0,$e0 dcb $00,$20,$40,$60,$80,$a0,$c0,$e0 color_pallete: dcb $01,$02,$03,$04,$05,$06,$07,$08,$09,$0a
Result of the code:
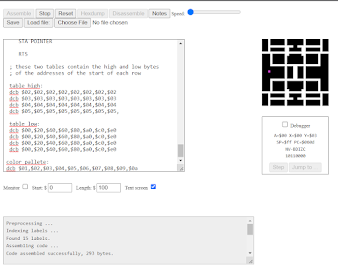
What have I learnt from this lab?
Even when the code is functional, it may have mistakes. It is preferable to think about which components should be fixed or tested before developing the code. Also, run numerous tests to identify where the issues are coming from.
Comments
Post a Comment